Centering a div (for back-end devs 😅)
Don't worry, I gotcha.
Honestly, I thought this was a joke. Until a couple days ago my friend told me it's actually hard for him. So here we are. Gaofan, this one is for you.
You are right, it's not that simple sometimes.
Don't want to add to your pain, but there are multiple ways to center a div. You can center it horizontally, vertically, or both. You can center it in the viewport, or in a parent element. You can center it using flexbox, or grid, with CSS, or JavaScript (or even with table-cell, but let's not go there).
Centering using margin
This is the simplest way to center a div. It works with block level elements likes divs, paragraphs, headings. You can take a look at the list of block level elements here.
Let's say you have a div
with a class
of box, and you want to center it horizontally. You can set the margin
property to auto
and it will calculate the margin on the left and right side of the element to center it for you.
.box { margin: auto; }
Centered with margin
Centering using flexbox
Trust me, flexbox is your best friend. Once you get the hang of it, you will use it for everything. And I mean everything. It can be used to center elements both horizontally — and vertically |. But this time we apply it to the parent element.
<divclass="parent">
<divclass="box"></div>
</div>
.parent { display: flex;justify-content: center;align-items: center; }
Centered with flexbox ✨
Centering using grid
Grid is another great way to center elements. It's a bit more complicated than flexbox, but centering with it is a breeze. It's also applied to the parent element and so we can use the same html structure.
.parent { display: grid;place-items: center; }
Centered with grid 🪟
Combining absolute positioning and transform
This is a bit of a hack, but it works. You can use position: absolute
to position the element in the center of the parent element. If we set the top
and left
properties to 50%
the top left corner of the element will be in the center of the parent element. The white dot is at the center of the parent element for reference.
.parent { position: relative; }
.box { position: absolute;top: 50%;left: 50%; }
Then you can use transform: translate(-50%, -50%)
to move the element back by 50% of its own width
and height
. The transform
property is relative to the element itself, not the parent element. This will center the element. And if you know the width and height of the element, you can use half of them too if you want. This box is 40px by 64px, so we can use transform: translate(-20px, -32px)
to center it.
.parent { position: absolute;top: 50%;left: 50%;transform: translate(-50%, -50%);/*transform: translate(-20px, -32px);*/ }
Obviously, there are other ways to center elements. But I believe as a back-end developer, you will be fine with these. I hope you won't have to use them too often. 🤐
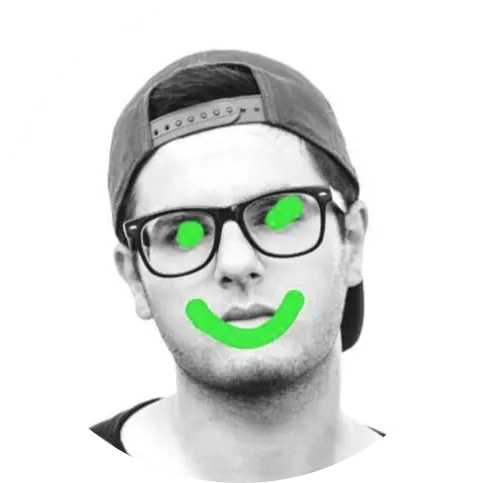